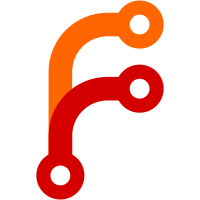
Since commit e65d1e69e8
there is no longer an
io.FileIO class, so this option is no longer needed.
This option also controlled whether or not files supported being opened in
binary mode (eg 'rb'), and could, if disabled, lead to confusion as to why
opening a file in binary mode silently did the wrong thing (it would just
open in text mode if MICROPY_PY_IO_FILEIO was disabled).
The various VFS implementations (POSIX, FAT, LFS) were the only places
where enabling this option made a difference, and in almost all cases where
one of these filesystems were enabled, MICROPY_PY_IO_FILEIO was also
enabled. So it makes sense to just unconditionally enable this feature
(ability to open a file in binary mode) in all cases, and so just remove
this config option altogether. That makes configuration simpler and means
binary file support always exists (and opening a file in binary mode is
arguably more fundamental than opening in text mode, so if anything should
be configurable then it should be the ability to open in text mode).
Signed-off-by: Damien George <damien@micropython.org>
123 lines
4.8 KiB
C
123 lines
4.8 KiB
C
/*
|
|
* This file is part of the MicroPython project, http://micropython.org/
|
|
*
|
|
* The MIT License (MIT)
|
|
*
|
|
* Copyright (c) 2015 Damien P. George
|
|
*
|
|
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
|
* of this software and associated documentation files (the "Software"), to deal
|
|
* in the Software without restriction, including without limitation the rights
|
|
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
|
* copies of the Software, and to permit persons to whom the Software is
|
|
* furnished to do so, subject to the following conditions:
|
|
*
|
|
* The above copyright notice and this permission notice shall be included in
|
|
* all copies or substantial portions of the Software.
|
|
*
|
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
|
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
|
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
|
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
|
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
|
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
|
* THE SOFTWARE.
|
|
*/
|
|
|
|
// options to control how MicroPython is built
|
|
|
|
#define MICROPY_ALLOC_PATH_MAX (PATH_MAX)
|
|
#define MICROPY_ENABLE_GC (1)
|
|
#define MICROPY_ENABLE_FINALISER (0)
|
|
#define MICROPY_STACK_CHECK (0)
|
|
#define MICROPY_COMP_CONST (0)
|
|
#define MICROPY_MEM_STATS (0)
|
|
#define MICROPY_DEBUG_PRINTERS (0)
|
|
#define MICROPY_READER_POSIX (1)
|
|
#define MICROPY_KBD_EXCEPTION (1)
|
|
#define MICROPY_HELPER_REPL (1)
|
|
#define MICROPY_HELPER_LEXER_UNIX (1)
|
|
#define MICROPY_ENABLE_SOURCE_LINE (0)
|
|
#define MICROPY_ERROR_REPORTING (MICROPY_ERROR_REPORTING_TERSE)
|
|
#define MICROPY_WARNINGS (0)
|
|
#define MICROPY_ENABLE_EMERGENCY_EXCEPTION_BUF (0)
|
|
#define MICROPY_FLOAT_IMPL (MICROPY_FLOAT_IMPL_NONE)
|
|
#define MICROPY_LONGINT_IMPL (MICROPY_LONGINT_IMPL_NONE)
|
|
#define MICROPY_STREAMS_NON_BLOCK (0)
|
|
#define MICROPY_OPT_COMPUTED_GOTO (0)
|
|
#define MICROPY_CAN_OVERRIDE_BUILTINS (0)
|
|
#define MICROPY_BUILTIN_METHOD_CHECK_SELF_ARG (0)
|
|
#define MICROPY_CPYTHON_COMPAT (0)
|
|
#define MICROPY_PY_BUILTINS_BYTEARRAY (0)
|
|
#define MICROPY_PY_BUILTINS_MEMORYVIEW (0)
|
|
#define MICROPY_PY_BUILTINS_COMPILE (0)
|
|
#define MICROPY_PY_BUILTINS_ENUMERATE (0)
|
|
#define MICROPY_PY_BUILTINS_FILTER (0)
|
|
#define MICROPY_PY_BUILTINS_FROZENSET (0)
|
|
#define MICROPY_PY_BUILTINS_REVERSED (0)
|
|
#define MICROPY_PY_BUILTINS_SET (0)
|
|
#define MICROPY_PY_BUILTINS_SLICE (0)
|
|
#define MICROPY_PY_BUILTINS_STR_UNICODE (0)
|
|
#define MICROPY_PY_BUILTINS_PROPERTY (0)
|
|
#define MICROPY_PY_BUILTINS_MIN_MAX (0)
|
|
#define MICROPY_PY___FILE__ (0)
|
|
#define MICROPY_PY_MICROPYTHON_MEM_INFO (0)
|
|
#define MICROPY_PY_GC (0)
|
|
#define MICROPY_PY_GC_COLLECT_RETVAL (0)
|
|
#define MICROPY_PY_ARRAY (0)
|
|
#define MICROPY_PY_COLLECTIONS (0)
|
|
#define MICROPY_PY_MATH (0)
|
|
#define MICROPY_PY_CMATH (0)
|
|
#define MICROPY_PY_IO (0)
|
|
#define MICROPY_PY_STRUCT (0)
|
|
#define MICROPY_PY_SYS (1)
|
|
#define MICROPY_PY_SYS_EXIT (0)
|
|
#define MICROPY_PY_SYS_PLATFORM "linux"
|
|
#define MICROPY_PY_SYS_MAXSIZE (0)
|
|
#define MICROPY_PY_SYS_PATH_DEFAULT ".frozen"
|
|
#define MICROPY_PY_SYS_STDFILES (0)
|
|
#define MICROPY_PY_CMATH (0)
|
|
#define MICROPY_PY_UCTYPES (0)
|
|
#define MICROPY_PY_UZLIB (0)
|
|
#define MICROPY_PY_UJSON (0)
|
|
#define MICROPY_PY_UOS (1)
|
|
#define MICROPY_PY_URE (0)
|
|
#define MICROPY_PY_UHEAPQ (0)
|
|
#define MICROPY_PY_UHASHLIB (0)
|
|
#define MICROPY_PY_UBINASCII (0)
|
|
|
|
//////////////////////////////////////////
|
|
// Do not change anything beyond this line
|
|
//////////////////////////////////////////
|
|
|
|
#if !(defined(MICROPY_GCREGS_SETJMP) || defined(__x86_64__) || defined(__i386__) || defined(__thumb2__) || defined(__thumb__) || defined(__arm__))
|
|
// Fall back to setjmp() implementation for discovery of GC pointers in registers.
|
|
#define MICROPY_GCREGS_SETJMP (1)
|
|
#endif
|
|
|
|
// type definitions for the specific machine
|
|
|
|
#ifdef __LP64__
|
|
typedef long mp_int_t; // must be pointer size
|
|
typedef unsigned long mp_uint_t; // must be pointer size
|
|
#else
|
|
// These are definitions for machines where sizeof(int) == sizeof(void*),
|
|
// regardless for actual size.
|
|
typedef int mp_int_t; // must be pointer size
|
|
typedef unsigned int mp_uint_t; // must be pointer size
|
|
#endif
|
|
|
|
// Cannot include <sys/types.h>, as it may lead to symbol name clashes
|
|
#if _FILE_OFFSET_BITS == 64 && !defined(__LP64__)
|
|
typedef long long mp_off_t;
|
|
#else
|
|
typedef long mp_off_t;
|
|
#endif
|
|
|
|
// We need to provide a declaration/definition of alloca()
|
|
#ifdef __FreeBSD__
|
|
#include <stdlib.h>
|
|
#else
|
|
#include <alloca.h>
|
|
#endif
|