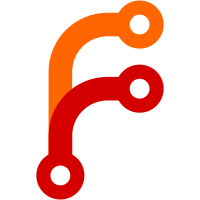
According to the OpenBSD man page, "A Replacement Call for Random". It offers high quality random numbers derived from input data obtained by the OpenBSD specific getentropy() system call which is declared in <unistd.h> and must be implemented for each Newlib port externally. The arc4random() functions are used for example in LibreSSL and OpenSSH. Cygwin provides currently its own implementation of the arc4random family. Maybe it makes sense to use this getentropy() implementation: http://cvsweb.openbsd.org/cgi-bin/cvsweb/src/lib/libcrypto/crypto/getentropy_win.c?rev=1.4&content-type=text/x-cvsweb-markup * libc/include/stdlib.h (arc4random): Declare if __BSD_VISIBLE. (arc4random_buf): Likewise. (arc4random_uniform): Likewise. * libc/include/sys/unistd.h (getentropy): Likewise. * libc/include/machine/_arc4random.h: New file. * libc/stdlib/arc4random.c: Likewise. * libc/stdlib/arc4random.h: Likewise. * libc/stdlib/arc4random_uniform.c: Likewise. * libc/stdlib/chacha_private.h: Likewise. * libc/sys/rtems/include/machine/_arc4random.h: Likewise. * libc/stdlib/Makefile.am (EXTENDED_SOURCES): Add arc4random.c and arc4random_uniform.c. * libc/stdlib/Makefile.in: Regenerate.
57 lines
1.8 KiB
C
57 lines
1.8 KiB
C
/* $OpenBSD: arc4random_uniform.c,v 1.1 2014/07/12 13:24:54 deraadt Exp $ */
|
|
|
|
/*
|
|
* Copyright (c) 2008, Damien Miller <djm@openbsd.org>
|
|
*
|
|
* Permission to use, copy, modify, and distribute this software for any
|
|
* purpose with or without fee is hereby granted, provided that the above
|
|
* copyright notice and this permission notice appear in all copies.
|
|
*
|
|
* THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
|
|
* WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
|
|
* MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
|
|
* ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
|
|
* WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
|
|
* ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF
|
|
* OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
|
|
*/
|
|
|
|
#include <sys/types.h>
|
|
#include <stdlib.h>
|
|
|
|
/*
|
|
* Calculate a uniformly distributed random number less than upper_bound
|
|
* avoiding "modulo bias".
|
|
*
|
|
* Uniformity is achieved by generating new random numbers until the one
|
|
* returned is outside the range [0, 2**32 % upper_bound). This
|
|
* guarantees the selected random number will be inside
|
|
* [2**32 % upper_bound, 2**32) which maps back to [0, upper_bound)
|
|
* after reduction modulo upper_bound.
|
|
*/
|
|
uint32_t
|
|
arc4random_uniform(uint32_t upper_bound)
|
|
{
|
|
uint32_t r, min;
|
|
|
|
if (upper_bound < 2)
|
|
return 0;
|
|
|
|
/* 2**32 % x == (2**32 - x) % x */
|
|
min = -upper_bound % upper_bound;
|
|
|
|
/*
|
|
* This could theoretically loop forever but each retry has
|
|
* p > 0.5 (worst case, usually far better) of selecting a
|
|
* number inside the range we need, so it should rarely need
|
|
* to re-roll.
|
|
*/
|
|
for (;;) {
|
|
r = arc4random();
|
|
if (r >= min)
|
|
break;
|
|
}
|
|
|
|
return r % upper_bound;
|
|
}
|