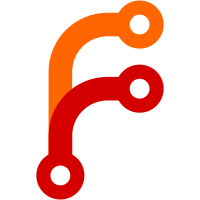
- Remove charset parameter from low level __foo_wctomb/__foo_mbtowc calls. - Instead, create array of function for ISO and Windows codepages to point to function which does not require to evaluate the charset string on each call. Create matching helper functions. I.e., __iso_wctomb, __iso_mbtowc, __cp_wctomb and __cp_mbtowc are functions returning the right function pointer now. - Create __WCTOMB/__MBTOWC macros utilizing per-reent locale and replace calls to __wctomb/__mbtowc with calls to __WCTOMB/__MBTOWC. - Drop global __wctomb/__mbtowc vars. - Utilize aforementioned changes in Cygwin to get rid of charset in other, calling functions and simplify the code. - In Cygwin restrict global cygheap locale info to the job performed by internal_setlocale. Use UTF-8 instead of ASCII on the fly in internal conversion functions. - In Cygwin dll_entry, make sure to initialize a TLS area with a NULL _REENT->_locale pointer. Add comment to explain why. Signed-off by: Corinna Vinschen <corinna@vinschen.de>
33 lines
512 B
C
33 lines
512 B
C
#include <wchar.h>
|
|
#include <stdlib.h>
|
|
#include <stdio.h>
|
|
#include <reent.h>
|
|
#include <string.h>
|
|
#include "local.h"
|
|
|
|
wint_t
|
|
btowc (int c)
|
|
{
|
|
mbstate_t mbs;
|
|
int retval = 0;
|
|
wchar_t pwc;
|
|
unsigned char b;
|
|
|
|
if (c == EOF)
|
|
return WEOF;
|
|
|
|
b = (unsigned char)c;
|
|
|
|
/* Put mbs in initial state. */
|
|
memset (&mbs, '\0', sizeof (mbs));
|
|
|
|
_REENT_CHECK_MISC(_REENT);
|
|
|
|
retval = __MBTOWC (_REENT, &pwc, (const char *) &b, 1, &mbs);
|
|
|
|
if (retval != 0 && retval != 1)
|
|
return WEOF;
|
|
|
|
return (wint_t)pwc;
|
|
}
|