forked from Lephenixnoir/PythonExtra
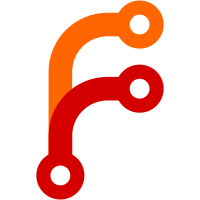
This commit implements a more complete replication of CPython's behaviour for equality and inequality testing of objects. This addresses the issues discussed in #5382 and a few other inconsistencies. Improvements over the old code include: - Support for returning non-boolean results from comparisons (as used by numpy and others). - Support for non-reflexive equality tests. - Preferential use of __ne__ methods and MP_BINARY_OP_NOT_EQUAL binary operators for inequality tests, when available. - Fallback to op2 == op1 or op2 != op1 when op1 does not implement the (in)equality operators. The scheme here makes use of a new flag, MP_TYPE_FLAG_NEEDS_FULL_EQ_TEST, in the flags word of mp_obj_type_t to indicate if various shortcuts can or cannot be used when performing equality and inequality tests. Currently four built-in classes have the flag set: float and complex are non-reflexive (since nan != nan) while bytearray and frozenszet instances can equal other builtin class instances (bytes and set respectively). The flag is also set for any new class defined by the user. This commit also includes a more comprehensive set of tests for the behaviour of (in)equality operators implemented in special methods.
597 lines
20 KiB
C
597 lines
20 KiB
C
/*
|
|
* This file is part of the MicroPython project, http://micropython.org/
|
|
*
|
|
* The MIT License (MIT)
|
|
*
|
|
* Copyright (c) 2013, 2014 Damien P. George
|
|
*
|
|
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
|
* of this software and associated documentation files (the "Software"), to deal
|
|
* in the Software without restriction, including without limitation the rights
|
|
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
|
* copies of the Software, and to permit persons to whom the Software is
|
|
* furnished to do so, subject to the following conditions:
|
|
*
|
|
* The above copyright notice and this permission notice shall be included in
|
|
* all copies or substantial portions of the Software.
|
|
*
|
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
|
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
|
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
|
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
|
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
|
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
|
* THE SOFTWARE.
|
|
*/
|
|
|
|
#include <stdint.h>
|
|
#include <stdio.h>
|
|
#include <stdarg.h>
|
|
#include <assert.h>
|
|
|
|
#include "py/obj.h"
|
|
#include "py/objtype.h"
|
|
#include "py/objint.h"
|
|
#include "py/objstr.h"
|
|
#include "py/runtime.h"
|
|
#include "py/stackctrl.h"
|
|
#include "py/stream.h" // for mp_obj_print
|
|
|
|
const mp_obj_type_t *mp_obj_get_type(mp_const_obj_t o_in) {
|
|
#if MICROPY_OBJ_IMMEDIATE_OBJS && MICROPY_OBJ_REPR == MICROPY_OBJ_REPR_A
|
|
|
|
if (mp_obj_is_obj(o_in)) {
|
|
const mp_obj_base_t *o = MP_OBJ_TO_PTR(o_in);
|
|
return o->type;
|
|
} else {
|
|
static const mp_obj_type_t *const types[] = {
|
|
NULL, &mp_type_int, &mp_type_str, &mp_type_int,
|
|
NULL, &mp_type_int, &mp_type_NoneType, &mp_type_int,
|
|
NULL, &mp_type_int, &mp_type_str, &mp_type_int,
|
|
NULL, &mp_type_int, &mp_type_bool, &mp_type_int,
|
|
};
|
|
return types[(uintptr_t)o_in & 0xf];
|
|
}
|
|
|
|
#elif MICROPY_OBJ_IMMEDIATE_OBJS && MICROPY_OBJ_REPR == MICROPY_OBJ_REPR_C
|
|
|
|
if (mp_obj_is_small_int(o_in)) {
|
|
return &mp_type_int;
|
|
} else if (mp_obj_is_obj(o_in)) {
|
|
const mp_obj_base_t *o = MP_OBJ_TO_PTR(o_in);
|
|
return o->type;
|
|
#if MICROPY_PY_BUILTINS_FLOAT
|
|
} else if ((((mp_uint_t)(o_in)) & 0xff800007) != 0x00000006) {
|
|
return &mp_type_float;
|
|
#endif
|
|
} else {
|
|
static const mp_obj_type_t *const types[] = {
|
|
&mp_type_str, &mp_type_NoneType, &mp_type_str, &mp_type_bool,
|
|
};
|
|
return types[((uintptr_t)o_in >> 3) & 3];
|
|
}
|
|
|
|
#else
|
|
|
|
if (mp_obj_is_small_int(o_in)) {
|
|
return &mp_type_int;
|
|
} else if (mp_obj_is_qstr(o_in)) {
|
|
return &mp_type_str;
|
|
#if MICROPY_PY_BUILTINS_FLOAT && ( \
|
|
MICROPY_OBJ_REPR == MICROPY_OBJ_REPR_C || MICROPY_OBJ_REPR == MICROPY_OBJ_REPR_D)
|
|
} else if (mp_obj_is_float(o_in)) {
|
|
return &mp_type_float;
|
|
#endif
|
|
#if MICROPY_OBJ_IMMEDIATE_OBJS
|
|
} else if (mp_obj_is_immediate_obj(o_in)) {
|
|
static const mp_obj_type_t *const types[2] = {&mp_type_NoneType, &mp_type_bool};
|
|
return types[MP_OBJ_IMMEDIATE_OBJ_VALUE(o_in) & 1];
|
|
#endif
|
|
} else {
|
|
const mp_obj_base_t *o = MP_OBJ_TO_PTR(o_in);
|
|
return o->type;
|
|
}
|
|
|
|
#endif
|
|
}
|
|
|
|
const char *mp_obj_get_type_str(mp_const_obj_t o_in) {
|
|
return qstr_str(mp_obj_get_type(o_in)->name);
|
|
}
|
|
|
|
void mp_obj_print_helper(const mp_print_t *print, mp_obj_t o_in, mp_print_kind_t kind) {
|
|
// There can be data structures nested too deep, or just recursive
|
|
MP_STACK_CHECK();
|
|
#ifndef NDEBUG
|
|
if (o_in == MP_OBJ_NULL) {
|
|
mp_print_str(print, "(nil)");
|
|
return;
|
|
}
|
|
#endif
|
|
const mp_obj_type_t *type = mp_obj_get_type(o_in);
|
|
if (type->print != NULL) {
|
|
type->print((mp_print_t*)print, o_in, kind);
|
|
} else {
|
|
mp_printf(print, "<%q>", type->name);
|
|
}
|
|
}
|
|
|
|
void mp_obj_print(mp_obj_t o_in, mp_print_kind_t kind) {
|
|
mp_obj_print_helper(MP_PYTHON_PRINTER, o_in, kind);
|
|
}
|
|
|
|
// helper function to print an exception with traceback
|
|
void mp_obj_print_exception(const mp_print_t *print, mp_obj_t exc) {
|
|
if (mp_obj_is_exception_instance(exc)) {
|
|
size_t n, *values;
|
|
mp_obj_exception_get_traceback(exc, &n, &values);
|
|
if (n > 0) {
|
|
assert(n % 3 == 0);
|
|
mp_print_str(print, "Traceback (most recent call last):\n");
|
|
for (int i = n - 3; i >= 0; i -= 3) {
|
|
#if MICROPY_ENABLE_SOURCE_LINE
|
|
mp_printf(print, " File \"%q\", line %d", values[i], (int)values[i + 1]);
|
|
#else
|
|
mp_printf(print, " File \"%q\"", values[i]);
|
|
#endif
|
|
// the block name can be NULL if it's unknown
|
|
qstr block = values[i + 2];
|
|
if (block == MP_QSTRnull) {
|
|
mp_print_str(print, "\n");
|
|
} else {
|
|
mp_printf(print, ", in %q\n", block);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
mp_obj_print_helper(print, exc, PRINT_EXC);
|
|
mp_print_str(print, "\n");
|
|
}
|
|
|
|
bool mp_obj_is_true(mp_obj_t arg) {
|
|
if (arg == mp_const_false) {
|
|
return 0;
|
|
} else if (arg == mp_const_true) {
|
|
return 1;
|
|
} else if (arg == mp_const_none) {
|
|
return 0;
|
|
} else if (mp_obj_is_small_int(arg)) {
|
|
if (arg == MP_OBJ_NEW_SMALL_INT(0)) {
|
|
return 0;
|
|
} else {
|
|
return 1;
|
|
}
|
|
} else {
|
|
const mp_obj_type_t *type = mp_obj_get_type(arg);
|
|
if (type->unary_op != NULL) {
|
|
mp_obj_t result = type->unary_op(MP_UNARY_OP_BOOL, arg);
|
|
if (result != MP_OBJ_NULL) {
|
|
return result == mp_const_true;
|
|
}
|
|
}
|
|
|
|
mp_obj_t len = mp_obj_len_maybe(arg);
|
|
if (len != MP_OBJ_NULL) {
|
|
// obj has a length, truth determined if len != 0
|
|
return len != MP_OBJ_NEW_SMALL_INT(0);
|
|
} else {
|
|
// any other obj is true per Python semantics
|
|
return 1;
|
|
}
|
|
}
|
|
}
|
|
|
|
bool mp_obj_is_callable(mp_obj_t o_in) {
|
|
const mp_call_fun_t call = mp_obj_get_type(o_in)->call;
|
|
if (call != mp_obj_instance_call) {
|
|
return call != NULL;
|
|
}
|
|
return mp_obj_instance_is_callable(o_in);
|
|
}
|
|
|
|
// This function implements the '==' and '!=' operators.
|
|
//
|
|
// From the Python language reference:
|
|
// (https://docs.python.org/3/reference/expressions.html#not-in)
|
|
// "The objects need not have the same type. If both are numbers, they are converted
|
|
// to a common type. Otherwise, the == and != operators always consider objects of
|
|
// different types to be unequal."
|
|
//
|
|
// This means that False==0 and True==1 are true expressions.
|
|
//
|
|
// Furthermore, from the v3.4.2 code for object.c: "Practical amendments: If rich
|
|
// comparison returns NotImplemented, == and != are decided by comparing the object
|
|
// pointer."
|
|
mp_obj_t mp_obj_equal_not_equal(mp_binary_op_t op, mp_obj_t o1, mp_obj_t o2) {
|
|
mp_obj_t local_true = (op == MP_BINARY_OP_NOT_EQUAL) ? mp_const_false : mp_const_true;
|
|
mp_obj_t local_false = (op == MP_BINARY_OP_NOT_EQUAL) ? mp_const_true : mp_const_false;
|
|
int pass_number = 0;
|
|
|
|
// Shortcut for very common cases
|
|
if (o1 == o2 &&
|
|
(mp_obj_is_small_int(o1) || !(mp_obj_get_type(o1)->flags & MP_TYPE_FLAG_NEEDS_FULL_EQ_TEST))) {
|
|
return local_true;
|
|
}
|
|
|
|
// fast path for strings
|
|
if (mp_obj_is_str(o1)) {
|
|
if (mp_obj_is_str(o2)) {
|
|
// both strings, use special function
|
|
return mp_obj_str_equal(o1, o2) ? local_true : local_false;
|
|
#if MICROPY_PY_STR_BYTES_CMP_WARN
|
|
} else if (mp_obj_is_type(o2, &mp_type_bytes)) {
|
|
str_bytes_cmp:
|
|
mp_warning(MP_WARN_CAT(BytesWarning), "Comparison between bytes and str");
|
|
return local_false;
|
|
#endif
|
|
} else {
|
|
goto skip_one_pass;
|
|
}
|
|
#if MICROPY_PY_STR_BYTES_CMP_WARN
|
|
} else if (mp_obj_is_str(o2) && mp_obj_is_type(o1, &mp_type_bytes)) {
|
|
// o1 is not a string (else caught above), so the objects are not equal
|
|
goto str_bytes_cmp;
|
|
#endif
|
|
}
|
|
|
|
// fast path for small ints
|
|
if (mp_obj_is_small_int(o1)) {
|
|
if (mp_obj_is_small_int(o2)) {
|
|
// both SMALL_INT, and not equal if we get here
|
|
return local_false;
|
|
} else {
|
|
goto skip_one_pass;
|
|
}
|
|
}
|
|
|
|
// generic type, call binary_op(MP_BINARY_OP_EQUAL)
|
|
while (pass_number < 2) {
|
|
const mp_obj_type_t *type = mp_obj_get_type(o1);
|
|
// If a full equality test is not needed and the other object is a different
|
|
// type then we don't need to bother trying the comparison.
|
|
if (type->binary_op != NULL &&
|
|
((type->flags & MP_TYPE_FLAG_NEEDS_FULL_EQ_TEST) || mp_obj_get_type(o2) == type)) {
|
|
// CPython is asymmetric: it will try __eq__ if there's no __ne__ but not the
|
|
// other way around. If the class doesn't need a full test we can skip __ne__.
|
|
if (op == MP_BINARY_OP_NOT_EQUAL && (type->flags & MP_TYPE_FLAG_NEEDS_FULL_EQ_TEST)) {
|
|
mp_obj_t r = type->binary_op(MP_BINARY_OP_NOT_EQUAL, o1, o2);
|
|
if (r != MP_OBJ_NULL) {
|
|
return r;
|
|
}
|
|
}
|
|
|
|
// Try calling __eq__.
|
|
mp_obj_t r = type->binary_op(MP_BINARY_OP_EQUAL, o1, o2);
|
|
if (r != MP_OBJ_NULL) {
|
|
if (op == MP_BINARY_OP_EQUAL) {
|
|
return r;
|
|
} else {
|
|
return mp_obj_is_true(r) ? local_true : local_false;
|
|
}
|
|
}
|
|
}
|
|
|
|
skip_one_pass:
|
|
// Try the other way around if none of the above worked
|
|
++pass_number;
|
|
mp_obj_t temp = o1;
|
|
o1 = o2;
|
|
o2 = temp;
|
|
}
|
|
|
|
// equality not implemented, so fall back to pointer conparison
|
|
return (o1 == o2) ? local_true : local_false;
|
|
}
|
|
|
|
bool mp_obj_equal(mp_obj_t o1, mp_obj_t o2) {
|
|
return mp_obj_is_true(mp_obj_equal_not_equal(MP_BINARY_OP_EQUAL, o1, o2));
|
|
}
|
|
|
|
mp_int_t mp_obj_get_int(mp_const_obj_t arg) {
|
|
// This function essentially performs implicit type conversion to int
|
|
// Note that Python does NOT provide implicit type conversion from
|
|
// float to int in the core expression language, try some_list[1.0].
|
|
if (arg == mp_const_false) {
|
|
return 0;
|
|
} else if (arg == mp_const_true) {
|
|
return 1;
|
|
} else if (mp_obj_is_small_int(arg)) {
|
|
return MP_OBJ_SMALL_INT_VALUE(arg);
|
|
} else if (mp_obj_is_type(arg, &mp_type_int)) {
|
|
return mp_obj_int_get_checked(arg);
|
|
} else {
|
|
mp_obj_t res = mp_unary_op(MP_UNARY_OP_INT, (mp_obj_t)arg);
|
|
return mp_obj_int_get_checked(res);
|
|
}
|
|
}
|
|
|
|
mp_int_t mp_obj_get_int_truncated(mp_const_obj_t arg) {
|
|
if (mp_obj_is_int(arg)) {
|
|
return mp_obj_int_get_truncated(arg);
|
|
} else {
|
|
return mp_obj_get_int(arg);
|
|
}
|
|
}
|
|
|
|
// returns false if arg is not of integral type
|
|
// returns true and sets *value if it is of integral type
|
|
// can throw OverflowError if arg is of integral type, but doesn't fit in a mp_int_t
|
|
bool mp_obj_get_int_maybe(mp_const_obj_t arg, mp_int_t *value) {
|
|
if (arg == mp_const_false) {
|
|
*value = 0;
|
|
} else if (arg == mp_const_true) {
|
|
*value = 1;
|
|
} else if (mp_obj_is_small_int(arg)) {
|
|
*value = MP_OBJ_SMALL_INT_VALUE(arg);
|
|
} else if (mp_obj_is_type(arg, &mp_type_int)) {
|
|
*value = mp_obj_int_get_checked(arg);
|
|
} else {
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
|
|
#if MICROPY_PY_BUILTINS_FLOAT
|
|
bool mp_obj_get_float_maybe(mp_obj_t arg, mp_float_t *value) {
|
|
mp_float_t val;
|
|
|
|
if (arg == mp_const_false) {
|
|
val = 0;
|
|
} else if (arg == mp_const_true) {
|
|
val = 1;
|
|
} else if (mp_obj_is_small_int(arg)) {
|
|
val = MP_OBJ_SMALL_INT_VALUE(arg);
|
|
#if MICROPY_LONGINT_IMPL != MICROPY_LONGINT_IMPL_NONE
|
|
} else if (mp_obj_is_type(arg, &mp_type_int)) {
|
|
val = mp_obj_int_as_float_impl(arg);
|
|
#endif
|
|
} else if (mp_obj_is_float(arg)) {
|
|
val = mp_obj_float_get(arg);
|
|
} else {
|
|
return false;
|
|
}
|
|
|
|
*value = val;
|
|
return true;
|
|
}
|
|
|
|
mp_float_t mp_obj_get_float(mp_obj_t arg) {
|
|
mp_float_t val;
|
|
|
|
if (!mp_obj_get_float_maybe(arg, &val)) {
|
|
if (MICROPY_ERROR_REPORTING == MICROPY_ERROR_REPORTING_TERSE) {
|
|
mp_raise_TypeError("can't convert to float");
|
|
} else {
|
|
nlr_raise(mp_obj_new_exception_msg_varg(&mp_type_TypeError,
|
|
"can't convert %s to float", mp_obj_get_type_str(arg)));
|
|
}
|
|
}
|
|
|
|
return val;
|
|
}
|
|
|
|
#if MICROPY_PY_BUILTINS_COMPLEX
|
|
void mp_obj_get_complex(mp_obj_t arg, mp_float_t *real, mp_float_t *imag) {
|
|
if (arg == mp_const_false) {
|
|
*real = 0;
|
|
*imag = 0;
|
|
} else if (arg == mp_const_true) {
|
|
*real = 1;
|
|
*imag = 0;
|
|
} else if (mp_obj_is_small_int(arg)) {
|
|
*real = MP_OBJ_SMALL_INT_VALUE(arg);
|
|
*imag = 0;
|
|
#if MICROPY_LONGINT_IMPL != MICROPY_LONGINT_IMPL_NONE
|
|
} else if (mp_obj_is_type(arg, &mp_type_int)) {
|
|
*real = mp_obj_int_as_float_impl(arg);
|
|
*imag = 0;
|
|
#endif
|
|
} else if (mp_obj_is_float(arg)) {
|
|
*real = mp_obj_float_get(arg);
|
|
*imag = 0;
|
|
} else if (mp_obj_is_type(arg, &mp_type_complex)) {
|
|
mp_obj_complex_get(arg, real, imag);
|
|
} else {
|
|
if (MICROPY_ERROR_REPORTING == MICROPY_ERROR_REPORTING_TERSE) {
|
|
mp_raise_TypeError("can't convert to complex");
|
|
} else {
|
|
nlr_raise(mp_obj_new_exception_msg_varg(&mp_type_TypeError,
|
|
"can't convert %s to complex", mp_obj_get_type_str(arg)));
|
|
}
|
|
}
|
|
}
|
|
#endif
|
|
#endif
|
|
|
|
// note: returned value in *items may point to the interior of a GC block
|
|
void mp_obj_get_array(mp_obj_t o, size_t *len, mp_obj_t **items) {
|
|
if (mp_obj_is_type(o, &mp_type_tuple)) {
|
|
mp_obj_tuple_get(o, len, items);
|
|
} else if (mp_obj_is_type(o, &mp_type_list)) {
|
|
mp_obj_list_get(o, len, items);
|
|
} else {
|
|
if (MICROPY_ERROR_REPORTING == MICROPY_ERROR_REPORTING_TERSE) {
|
|
mp_raise_TypeError("expected tuple/list");
|
|
} else {
|
|
nlr_raise(mp_obj_new_exception_msg_varg(&mp_type_TypeError,
|
|
"object '%s' isn't a tuple or list", mp_obj_get_type_str(o)));
|
|
}
|
|
}
|
|
}
|
|
|
|
// note: returned value in *items may point to the interior of a GC block
|
|
void mp_obj_get_array_fixed_n(mp_obj_t o, size_t len, mp_obj_t **items) {
|
|
size_t seq_len;
|
|
mp_obj_get_array(o, &seq_len, items);
|
|
if (seq_len != len) {
|
|
if (MICROPY_ERROR_REPORTING == MICROPY_ERROR_REPORTING_TERSE) {
|
|
mp_raise_ValueError("tuple/list has wrong length");
|
|
} else {
|
|
nlr_raise(mp_obj_new_exception_msg_varg(&mp_type_ValueError,
|
|
"requested length %d but object has length %d", (int)len, (int)seq_len));
|
|
}
|
|
}
|
|
}
|
|
|
|
// is_slice determines whether the index is a slice index
|
|
size_t mp_get_index(const mp_obj_type_t *type, size_t len, mp_obj_t index, bool is_slice) {
|
|
mp_int_t i;
|
|
if (mp_obj_is_small_int(index)) {
|
|
i = MP_OBJ_SMALL_INT_VALUE(index);
|
|
} else if (!mp_obj_get_int_maybe(index, &i)) {
|
|
if (MICROPY_ERROR_REPORTING == MICROPY_ERROR_REPORTING_TERSE) {
|
|
mp_raise_TypeError("indices must be integers");
|
|
} else {
|
|
nlr_raise(mp_obj_new_exception_msg_varg(&mp_type_TypeError,
|
|
"%q indices must be integers, not %s",
|
|
type->name, mp_obj_get_type_str(index)));
|
|
}
|
|
}
|
|
|
|
if (i < 0) {
|
|
i += len;
|
|
}
|
|
if (is_slice) {
|
|
if (i < 0) {
|
|
i = 0;
|
|
} else if ((mp_uint_t)i > len) {
|
|
i = len;
|
|
}
|
|
} else {
|
|
if (i < 0 || (mp_uint_t)i >= len) {
|
|
if (MICROPY_ERROR_REPORTING == MICROPY_ERROR_REPORTING_TERSE) {
|
|
mp_raise_msg(&mp_type_IndexError, "index out of range");
|
|
} else {
|
|
nlr_raise(mp_obj_new_exception_msg_varg(&mp_type_IndexError,
|
|
"%q index out of range", type->name));
|
|
}
|
|
}
|
|
}
|
|
|
|
// By this point 0 <= i <= len and so fits in a size_t
|
|
return (size_t)i;
|
|
}
|
|
|
|
mp_obj_t mp_obj_id(mp_obj_t o_in) {
|
|
mp_int_t id = (mp_int_t)o_in;
|
|
if (!mp_obj_is_obj(o_in)) {
|
|
return mp_obj_new_int(id);
|
|
} else if (id >= 0) {
|
|
// Many OSes and CPUs have affinity for putting "user" memories
|
|
// into low half of address space, and "system" into upper half.
|
|
// We're going to take advantage of that and return small int
|
|
// (signed) for such "user" addresses.
|
|
return MP_OBJ_NEW_SMALL_INT(id);
|
|
} else {
|
|
// If that didn't work, well, let's return long int, just as
|
|
// a (big) positive value, so it will never clash with the range
|
|
// of small int returned in previous case.
|
|
return mp_obj_new_int_from_uint((mp_uint_t)id);
|
|
}
|
|
}
|
|
|
|
// will raise a TypeError if object has no length
|
|
mp_obj_t mp_obj_len(mp_obj_t o_in) {
|
|
mp_obj_t len = mp_obj_len_maybe(o_in);
|
|
if (len == MP_OBJ_NULL) {
|
|
if (MICROPY_ERROR_REPORTING == MICROPY_ERROR_REPORTING_TERSE) {
|
|
mp_raise_TypeError("object has no len");
|
|
} else {
|
|
nlr_raise(mp_obj_new_exception_msg_varg(&mp_type_TypeError,
|
|
"object of type '%s' has no len()", mp_obj_get_type_str(o_in)));
|
|
}
|
|
} else {
|
|
return len;
|
|
}
|
|
}
|
|
|
|
// may return MP_OBJ_NULL
|
|
mp_obj_t mp_obj_len_maybe(mp_obj_t o_in) {
|
|
if (
|
|
#if !MICROPY_PY_BUILTINS_STR_UNICODE
|
|
// It's simple - unicode is slow, non-unicode is fast
|
|
mp_obj_is_str(o_in) ||
|
|
#endif
|
|
mp_obj_is_type(o_in, &mp_type_bytes)) {
|
|
GET_STR_LEN(o_in, l);
|
|
return MP_OBJ_NEW_SMALL_INT(l);
|
|
} else {
|
|
const mp_obj_type_t *type = mp_obj_get_type(o_in);
|
|
if (type->unary_op != NULL) {
|
|
return type->unary_op(MP_UNARY_OP_LEN, o_in);
|
|
} else {
|
|
return MP_OBJ_NULL;
|
|
}
|
|
}
|
|
}
|
|
|
|
mp_obj_t mp_obj_subscr(mp_obj_t base, mp_obj_t index, mp_obj_t value) {
|
|
const mp_obj_type_t *type = mp_obj_get_type(base);
|
|
if (type->subscr != NULL) {
|
|
mp_obj_t ret = type->subscr(base, index, value);
|
|
if (ret != MP_OBJ_NULL) {
|
|
return ret;
|
|
}
|
|
// TODO: call base classes here?
|
|
}
|
|
if (value == MP_OBJ_NULL) {
|
|
if (MICROPY_ERROR_REPORTING == MICROPY_ERROR_REPORTING_TERSE) {
|
|
mp_raise_TypeError("object doesn't support item deletion");
|
|
} else {
|
|
nlr_raise(mp_obj_new_exception_msg_varg(&mp_type_TypeError,
|
|
"'%s' object doesn't support item deletion", mp_obj_get_type_str(base)));
|
|
}
|
|
} else if (value == MP_OBJ_SENTINEL) {
|
|
if (MICROPY_ERROR_REPORTING == MICROPY_ERROR_REPORTING_TERSE) {
|
|
mp_raise_TypeError("object isn't subscriptable");
|
|
} else {
|
|
nlr_raise(mp_obj_new_exception_msg_varg(&mp_type_TypeError,
|
|
"'%s' object isn't subscriptable", mp_obj_get_type_str(base)));
|
|
}
|
|
} else {
|
|
if (MICROPY_ERROR_REPORTING == MICROPY_ERROR_REPORTING_TERSE) {
|
|
mp_raise_TypeError("object doesn't support item assignment");
|
|
} else {
|
|
nlr_raise(mp_obj_new_exception_msg_varg(&mp_type_TypeError,
|
|
"'%s' object doesn't support item assignment", mp_obj_get_type_str(base)));
|
|
}
|
|
}
|
|
}
|
|
|
|
// Return input argument. Useful as .getiter for objects which are
|
|
// their own iterators, etc.
|
|
mp_obj_t mp_identity(mp_obj_t self) {
|
|
return self;
|
|
}
|
|
MP_DEFINE_CONST_FUN_OBJ_1(mp_identity_obj, mp_identity);
|
|
|
|
mp_obj_t mp_identity_getiter(mp_obj_t self, mp_obj_iter_buf_t *iter_buf) {
|
|
(void)iter_buf;
|
|
return self;
|
|
}
|
|
|
|
bool mp_get_buffer(mp_obj_t obj, mp_buffer_info_t *bufinfo, mp_uint_t flags) {
|
|
const mp_obj_type_t *type = mp_obj_get_type(obj);
|
|
if (type->buffer_p.get_buffer == NULL) {
|
|
return false;
|
|
}
|
|
int ret = type->buffer_p.get_buffer(obj, bufinfo, flags);
|
|
if (ret != 0) {
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
|
|
void mp_get_buffer_raise(mp_obj_t obj, mp_buffer_info_t *bufinfo, mp_uint_t flags) {
|
|
if (!mp_get_buffer(obj, bufinfo, flags)) {
|
|
mp_raise_TypeError("object with buffer protocol required");
|
|
}
|
|
}
|
|
|
|
mp_obj_t mp_generic_unary_op(mp_unary_op_t op, mp_obj_t o_in) {
|
|
switch (op) {
|
|
case MP_UNARY_OP_HASH: return MP_OBJ_NEW_SMALL_INT((mp_uint_t)o_in);
|
|
default: return MP_OBJ_NULL; // op not supported
|
|
}
|
|
}
|