forked from Lephenixnoir/PythonExtra
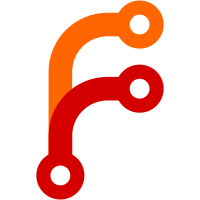
Now that constant tuples are supported in the parser, eg (1, True, "str"), it's a small step to allow anything that is a constant to be used with the pattern: from micropython import const X = const(obj) This commit makes the required changes to allow the following types of constants: from micropython import const _INT = const(123) _FLOAT = const(1.2) _COMPLEX = const(3.4j) _STR = const("str") _BYTES = const(b"bytes") _TUPLE = const((_INT, _STR, _BYTES)) _TUPLE2 = const((None, False, True, ..., (), _TUPLE)) Prior to this, only integers could be used in const(...). Signed-off-by: Damien George <damien@micropython.org>
27 lines
651 B
Python
27 lines
651 B
Python
# Test constant optimisation, with full range of const types.
|
|
# This test will only work when MICROPY_COMP_CONST and MICROPY_COMP_CONST_TUPLE are enabled.
|
|
|
|
from micropython import const
|
|
|
|
_INT = const(123)
|
|
_STR = const("str")
|
|
_BYTES = const(b"bytes")
|
|
_TUPLE = const((_INT, _STR, _BYTES))
|
|
_TUPLE2 = const((None, False, True, ..., (), _TUPLE))
|
|
|
|
print(_INT)
|
|
print(_STR)
|
|
print(_BYTES)
|
|
print(_TUPLE)
|
|
print(_TUPLE2)
|
|
|
|
x = _TUPLE
|
|
print(x is _TUPLE)
|
|
print(x is (_INT, _STR, _BYTES))
|
|
|
|
print(hasattr(globals(), "_INT"))
|
|
print(hasattr(globals(), "_STR"))
|
|
print(hasattr(globals(), "_BYTES"))
|
|
print(hasattr(globals(), "_TUPLE"))
|
|
print(hasattr(globals(), "_TUPLE2"))
|